Software Development Best Practices
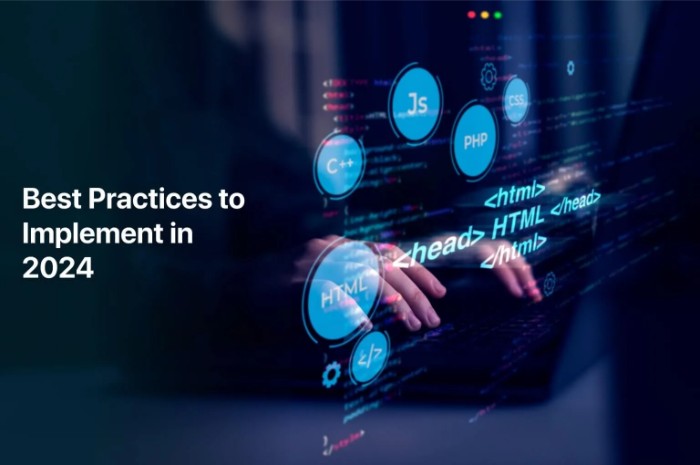
In the dynamic world of software development, adhering to best practices is essential for creating robust, efficient, and maintainable software. Best practices are guidelines, ethics, development standards, or ideas representing the most efficient course of action in a given business situation. Following these practices leads to improved code quality, efficiency, and easier maintenance. Principles such as DRY and YAGNI provide guidance for effective coding and feature development. Robust software is achieved through thorough testing, version control, and diligent code reviews.
At Netguru, we take software development best practices seriously. By adhering to these guidelines, we ensure the software and applications we create for our clients and their end users are well-designed, coded, robust, and thoroughly tested. Identifying and sharing these best practices nurtures a learning culture, fills knowledge gaps, enhances efficiency, enables better decision-making, and provides employees with an internal knowledge base. Ultimately, this leads to cost savings and time efficiency, as all team members are on the same page throughout the software development lifecycle.
Top 10 Software Development Best Practices
1. DRY Principle
The DRY principle stands for “Don’t Repeat Yourself.” Formulated in 1999 by Andy Hunt and Dave Thomas, it emphasizes that “every piece of knowledge must have a single, unambiguous, authoritative representation within a system.” This principle remains relevant today and should be applied to any piece of code you design. Avoiding knowledge duplication means business logic with a certain functionality should only appear once in your application. However, code duplication is acceptable when it refers to different business applications, as it helps prevent mistakes and reduces time consumption.
2. YAGNI Principle
YAGNI stands for “You Ain’t Gonna Need It.” This principle advises against writing code that isn’t needed immediately but might be useful in the future. The rationale is that the future may never come, or requirements may change, making the code obsolete. To avoid wasting time and resources, organize planning sessions and split big features into smaller, independent tasks. This prevents the release of half-finished code that needs to be completed later.
3. Testing Best Practices
Writing unit tests is one of the easiest ways to improve code quality. Unit tests tackle small, modular pieces of code, testing one functionality at a time. Consider different scenarios, including failure cases, and aim for 100% test coverage. Use libraries designed to calculate test coverage, such as Istanbul for Node.js, Coverage for Python, and Serenity or JCov for Java.
4. Version Control
Version control is crucial for tracking and managing changes to software code, especially when multiple developers are involved. Tools like Git and GitHub allow for simultaneous development without code conflicts. Version control systems also enable easy reversion to previous versions if mistakes occur. Other tools include CVS, SVN, and Mercurial.
5. Embrace AI Assistance
Leveraging AI tools can streamline software development processes. GitHub Copilot, an AI-driven code completion tool, enhances productivity by providing real-time code suggestions. This tool supports multiple programming languages and integrates seamlessly with popular IDEs like Visual Studio Code, helping developers write efficient code and learn new languages.
6. Pay Attention to Style Guides
Style guides make code more readable and enjoyable to work with. Using a linter tool, which serves as a static code analyzer, can improve code quality by showing where style can be improved and fixing minor issues automatically. Examples of linter tools include ESLint for JavaScript, RuboCop for Ruby, and Flake8 or Pylint for Python.
7. Naming Conventions
Proper naming conventions for variables, types, functions, and other entities in your source code and documentation enhance readability and refactoring ease. Meaningful names provide instant understanding of a function’s purpose, reducing guesswork and saving time. For example:
def c(a, b):
return a * b
def count_weekly_pay(hours_worked, hourly_pay_rate):return hours_worked * hourly_pay_rate
Both functions perform the same task, but the second example is more readable and self-explanatory.
8. Code Reviews
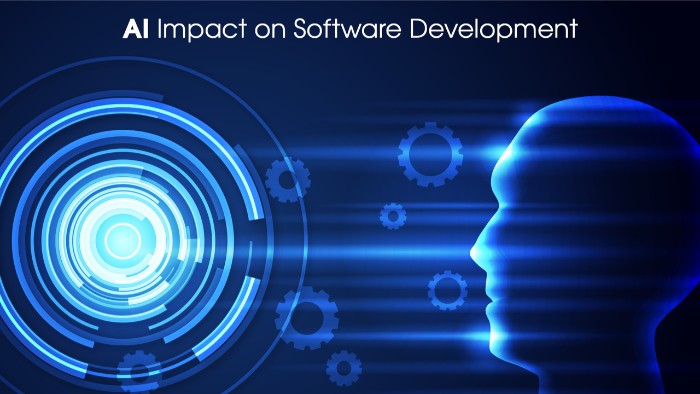
Software Development Best Practices in 2024
Regular code reviews ensure code quality, maintainability, and adherence to best practices. They provide opportunities for knowledge sharing and identifying potential issues early. Code reviews should be a collaborative process, focusing on constructive feedback and continuous improvement.
9. Continuous Integration and Continuous Deployment (CI/CD)
CI/CD practices automate the integration and deployment process, ensuring that code changes are continuously tested and deployed. This reduces the risk of integration issues and allows for faster delivery of features. Tools like Jenkins, Travis CI, and CircleCI can facilitate CI/CD processes.
10. Documentation
Comprehensive documentation is vital for maintaining and scaling software projects. It provides a reference for current and future developers, ensuring that the codebase is understandable and maintainable. Documentation should include code comments, README files, and detailed API documentation.
Following these best practices in software development ensures the creation of high-quality, efficient, and maintainable software. By implementing these guidelines, development teams can enhance collaboration, reduce errors, and deliver robust software solutions.